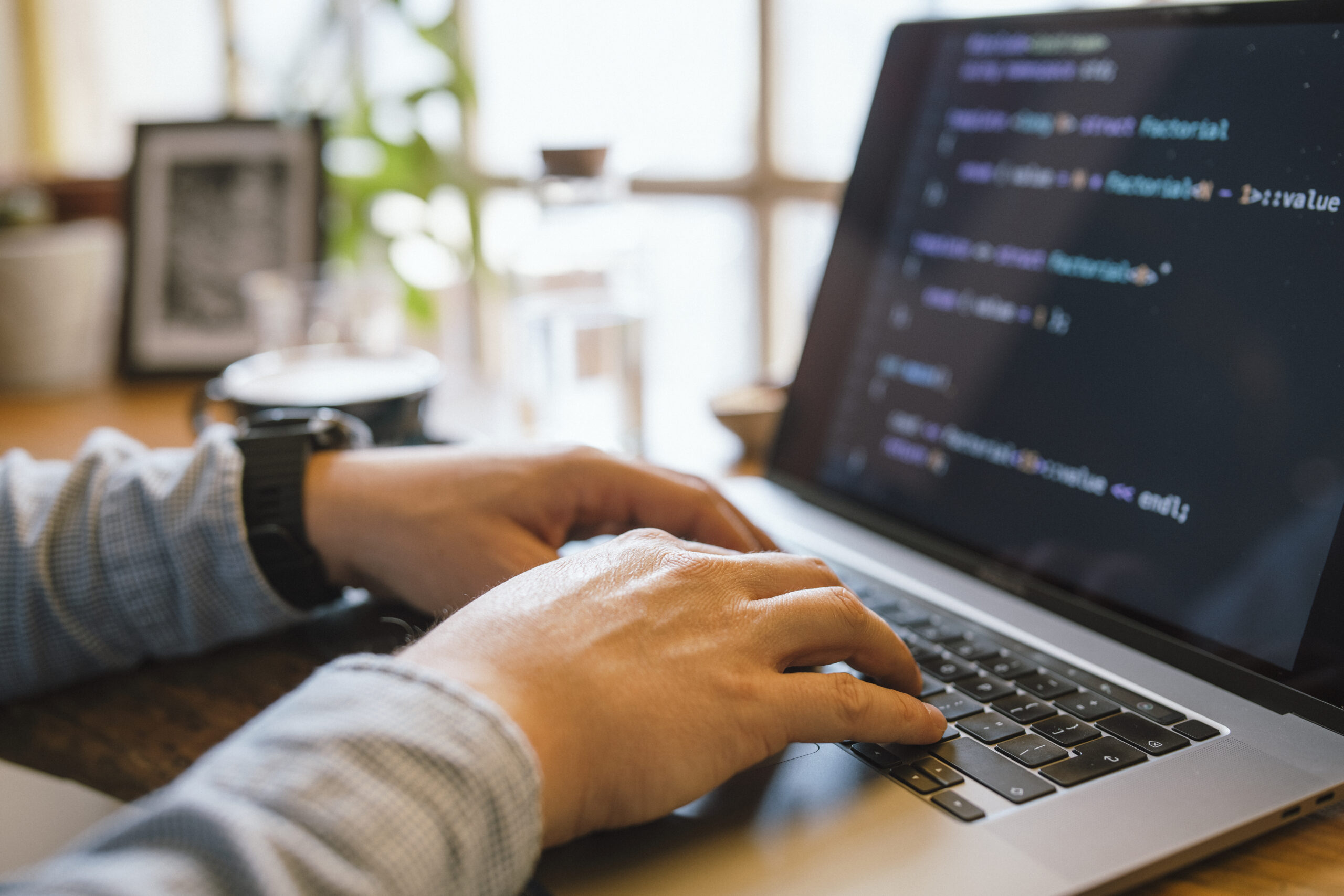
Debugging is One of the more important — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Here are quite a few procedures that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest techniques developers can elevate their debugging competencies is by mastering the applications they use on a daily basis. Even though creating code is one Element of progress, being aware of the best way to interact with it correctly for the duration of execution is equally vital. Modern-day advancement environments appear Outfitted with strong debugging capabilities — but many builders only scratch the surface area of what these instruments can do.
Choose, such as, an Built-in Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment enable you to set breakpoints, inspect the worth of variables at runtime, move by means of code line by line, and even modify code to the fly. When employed correctly, they Enable you to notice just how your code behaves during execution, and that is invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert frustrating UI troubles into workable tasks.
For backend or program-amount builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your equipment means going past default options and shortcuts — it’s about establishing an intimate familiarity with your growth natural environment to make sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you know your tools, the greater time you could expend resolving the particular challenge rather than fumbling through the process.
Reproduce the Problem
One of the most critical — and infrequently forgotten — techniques in productive debugging is reproducing the situation. Ahead of jumping in to the code or making guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of opportunity, often resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve gathered sufficient information and facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking technique states. If the issue seems intermittently, consider composing automatic exams that replicate the sting conditions or condition transitions included. These tests not just enable expose the issue but in addition reduce regressions Later on.
From time to time, The difficulty might be natural environment-unique — it might come about only on selected operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra effectively, test potential fixes safely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s in which builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers should master to take care of mistake messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in full. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper during the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate brought on it? These queries can guide your investigation and level you towards the responsible code.
It’s also handy to know the terminology with the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some faults are vague or generic, As well as in those circumstances, it’s important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details throughout development, INFO for typical gatherings (like profitable start off-ups), WARN for potential challenges that don’t split the application, Mistake for genuine troubles, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target important events, condition modifications, enter/output values, and demanding decision points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re especially worthwhile in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a nicely-imagined-out logging solution, you'll be able to lessen the time it will take to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This way of thinking allows stop working complicated concerns into manageable areas and observe clues logically to uncover the foundation cause.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer stories to piece collectively a clear image of what’s happening.
Subsequent, form hypotheses. Ask oneself: What could possibly be creating this behavior? Have any changes recently been built into the codebase? Has this situation transpired just before below click here similar circumstances? The target would be to slim down prospects and determine possible culprits.
Then, test your theories systematically. Seek to recreate the condition in the controlled atmosphere. If you suspect a certain operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code queries and let the effects direct you closer to the truth.
Spend close awareness to modest particulars. Bugs normally cover within the the very least anticipated places—just like a missing semicolon, an off-by-one particular error, or maybe a race problem. Be thorough and individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may perhaps conceal the actual difficulty, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can save time for foreseeable future issues and aid Many others realize your reasoning.
By wondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and turn out to be simpler at uncovering concealed issues in complicated programs.
Generate Tests
Creating assessments is among the simplest ways to boost your debugging capabilities and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that offers you confidence when creating adjustments for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Operating as expected. Any time a exam fails, you straight away know wherever to glance, appreciably minimizing time invested debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclusion-to-conclude tests into your workflow. These help be sure that a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in complex devices with several components or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To test a element correctly, you need to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can target correcting the bug and watch your examination go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing recreation into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this point out, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Power in addition to a clearer way of thinking. You could suddenly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that time to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially under restricted deadlines, but it really truly causes quicker and more practical debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing worthwhile when you go to the trouble to reflect and analyze what went Improper.
Start off by inquiring on your own a handful of vital questions once the bug is resolved: What triggered it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or knowing and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a superb routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular faults—which you could proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, many of the very best builders aren't those who write best code, but those who repeatedly learn from their problems.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging competencies requires time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at Whatever you do.